Asynchronous Comparison
Synchronous comparison is used when the response is obtained quickly and where the result file is returned as part of the response.
However in other cases asynchronous comparison is useful:
If there is a risk of HTTP timeout
When the comparison result is needed somewhere other than where the response is returned
Where progress, logging or resource consumption information is needed
When multiple comparisons can be started at once
XML/JSON Request
Whether using XML or JSON, an asynchronous comparison will use the async element to specify the optional values callback
and keepResult
.
callback
provides a callback URL that the REST service will make aGET
request to after the comparison is complete. This is detailed in Callbacks below.keepResult
is a boolean value indicating whether to keep the result file after reading the result output for the first time.
The response will consist of the created Job, and a Location header pointing to where you can poll the Job status.
Add the <async/> element to the body of the POST request
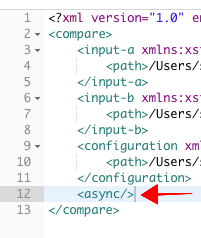
The response indicates that it has been accepted and gives the job number. In Postman, click on the link in the body of the response to get the status.
Body of Response Example
<job>
<jobId>855b6698-e2ad-45bc-bb51-9f4d8b9df715</jobId>
<jobStatus>QUEUED</jobStatus>
<totalNumberOfStages>0</totalNumberOfStages>
<currentStage>0</currentStage>
<links>
<link href="/api/xml-data-compare/v1/jobs/855b6698-e2ad-45bc-bb51-9f4d8b9df715"/>
</links>
</job>
Once on the GET for the Job status, hit Send to get the status. You can repeatedly click on the Send button to see the job progressing through the stages.
Job Status 'Processing'
<job>
<jobId>3180d315-fc13-42a4-a872-fca1126274b6</jobId>
<startTime>2019-02-07T13:50:58.528+0000</startTime>
<jobStatus>PROCESSING</jobStatus>
<phaseDescription>Comparison in progress</phaseDescription>
<totalNumberOfStages>23</totalNumberOfStages>
<currentStage>15</currentStage>
<links/>
</job>
Job Status 'Success'
<job>
<jobId>5e3e85ec-b1eb-45d8-9295-238767881e97</jobId>
<startTime>2019-02-07T13:55:32.484+0000</startTime>
<finishedTime>2019-02-07T13:55:44.249+0000</finishedTime>
<jobStatus>SUCCESS</jobStatus>
<output type="http">
<size>20409</size>
<uri>http://0.0.0.0:8080/api/xml-data-compare/v1/downloads/5e3e85ec-b1eb-45d8-9295-238767881e97</uri>
</output>
<totalNumberOfStages>23</totalNumberOfStages>
<currentStage>23</currentStage>
<links/>
</job>
Once the job has reached the final stage, with a status of Success, in Postman, the URI can be clicked to copy it to a new GET request. Click on Send to obtain the results.
Form Request
When using multipart/form-data, set the parameter async
to true.
You can also use the parameter callback
in a similar fashion to XML/JSON async requests.
.....
Content-Disposition: form-data; name= "async"
true
--boundary
Content-Disposition: form-data; name= "callback"
http: //www.example.com/comparison-callback
--boundary
.....
Jobs
For each async comparison a Job is created. The jobs can be read from the /jobs/{jobId}
endpoint. For example, you can poll for the Job's status:
Request
GET /api/xml-data-compare/v1/jobs/5e3e85ec-b1eb-45d8-9295-238767881e97
Job Model
The Job contains the following objects:
Object | Description |
---|---|
| |
| ISO 8601 timestamp when the comparison started. |
| ISO 8601 timestamp when the comparison finished. |
| An enumeration of the state of the comparison. States include:
|
| Giving the type, the size and the URI |
| A description of the phase of the job, including:
|
| Number of stages in the job, as an integer. |
| The current stage of the job. |
| HATEOAS links containing a A rel of "cancel" indicates you can use |
Deleting a Job
The queued or running jobs can be deleted. This is indicated through a HATEOAS link in the Job info when it is queued or running, for example:
Response (XML)
<job>
<jobId>930398ea-46e3-485a-aad4-ee4a268fd658</jobId>
<startTime>2019-02-26T15:14:48.165+0000</startTime>
<jobStatus>PROCESSING</jobStatus>
<phaseDescription>Comparison in progress</phaseDescription>
<totalNumberOfStages>10</totalNumberOfStages>
<currentStage>5</currentStage>
<links>
<link>
<rel>delete</rel>
<href>/api/xml-data-compare/v1/jobs/ed220cbd-1596-417c-a4ba-2c0ce67cc6ac</href>
</link>
</links>
</job>
Response (JSON)
{
"jobId": "930398ea-46e3-485a-aad4-ee4a268fd658",
"startTime": "2019-02-26T15:13:29.804+0000",
"jobStatus": "STARTED",
"totalNumberOfStages": 0,
"currentStage": 0,
"link": [
{
"rel": "delete",
"href": "/api/xml-data-compare/v1/jobs/930398ea-46e3-485a-aad4-ee4a268fd658"
}
]
}
It is invoked by using a DELETE
request to the specified Job:
Request
DELETE /api/xml-data-compare/v1/jobs/930398ea-46e3-485a-aad4-ee4a268fd658
The response will be the Job, note the jobStatus
is now DELETED.:
Response
<job>
<jobId>d278fecb-7883-4604-9d62-673f1e080402</jobId>
<startTime>2019-02-26T15:15:57.605+0000</startTime>
<jobStatus>DELETED</jobStatus>
<phaseDescription>Output processing in progress</phaseDescription>
<totalNumberOfStages>10</totalNumberOfStages>
<currentStage>5</currentStage>
<links/>
</job>
Response
{
"jobId": "7801447a-3407-4235-8220-362a31801a91",
"startTime": "2019-02-26T15:28:08.667+0000",
"finishedTime": "2019-02-26T15:28:25.223+0000",
"jobStatus": "DELETED",
"totalNumberOfStages": 10,
"currentStage": 5,
"link": []
}
Please not that the job does not exist once it is deleted.
Download result
A GET
request to the output
uri returned by successful job will then complete the asynchronous lifecycle:
Request
GET /api/xml-data-compare/v1/downloads/123456
Response
HTTP/1.1 200 OK
<root xmlns:deltaxml="http://deltaxml.com/ns/well-formed-delta-v1" deltaxml:deltaV2="A!=B">
<child deltaxml:deltaV2="A=B">...</child>
<child deltaxml:deltaV2="A!=B">...</child>
</root>
Note
If the POST request specified an output file location, the output file will be available at that location after a successful asynchronous comparison. A GET request from the downloads resource will merely provide the same file from that location.
Callbacks
An URI, such as http://myserver/callback, to a location which will be called when the comparison has successfully completed and the result is ready. It must accept GET HTTP Method.